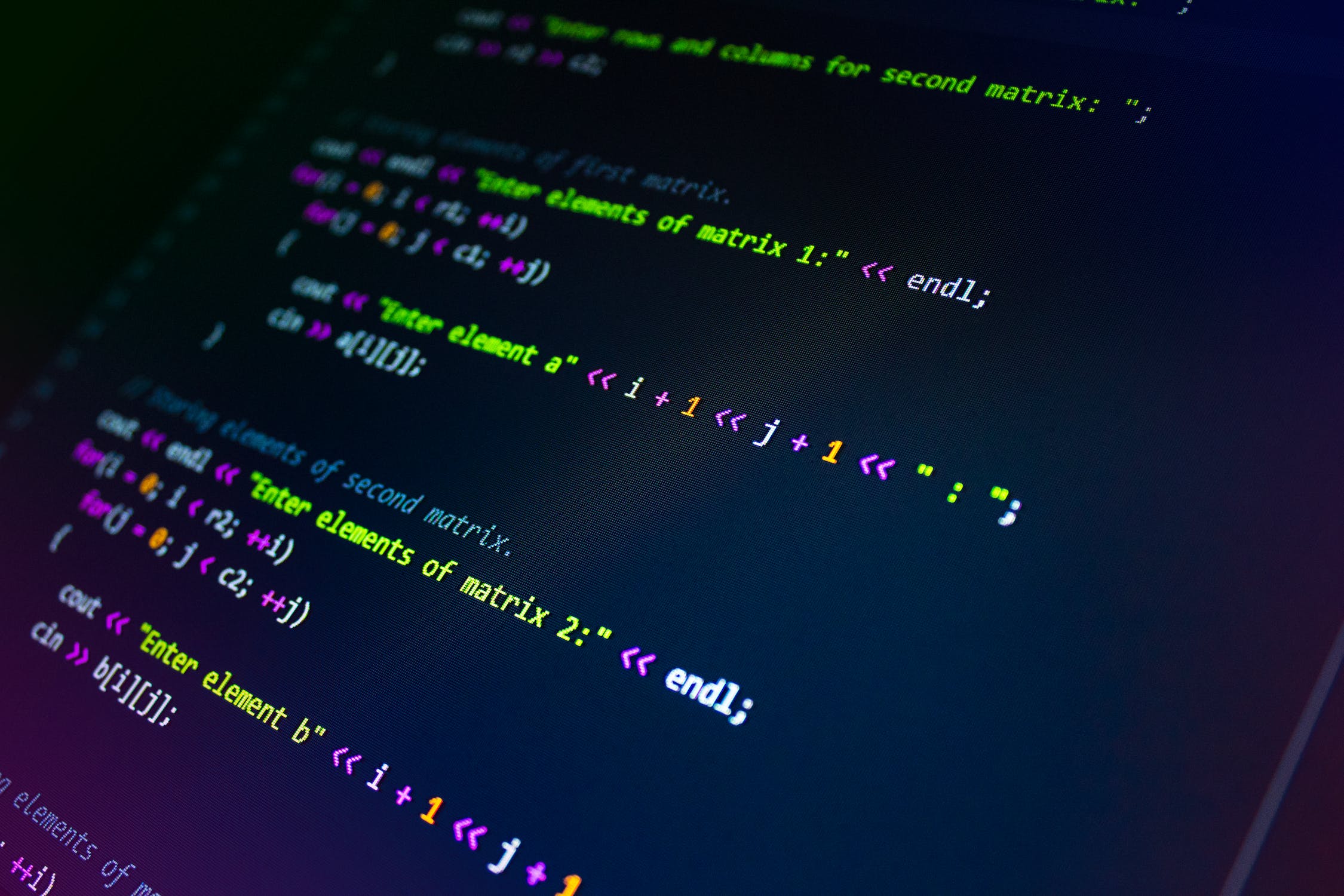
Exploring Prisma: A Comprehensive ORM for Backend Development
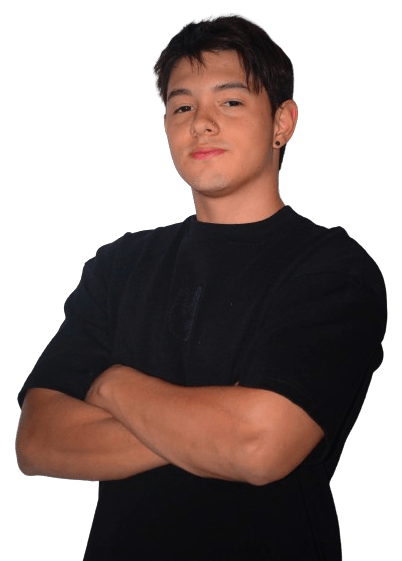
Exploring Prisma: A Comprehensive ORM for Backend Development
Introduction:
Prisma has risen to prominence as a powerful Object-Relational Mapping (ORM) tool, streamlining database access and manipulation in the backend development landscape. In this article, we’ll delve into the key features that make Prisma a compelling choice for building scalable, efficient, and maintainable backend applications.
1. Declarative Data Modeling:
Prisma adopts a declarative approach to data modeling, allowing developers to define the structure of their data using a simple and intuitive syntax. This not only enhances code readability but also accelerates the development process by minimizing the need for verbose configurations.
// Example of Declarative Data Modeling in Prisma
model User {
id Int @id @default(autoincrement())
name String
email String @unique
posts Post[]
}
model Post {
id Int @id @default(autoincrement())
title String
body String
userId Int
user User @relation(fields: [userId], references: [id])
}
2. Type-Safe Queries:
Prisma offers type-safe queries, leveraging the TypeScript language to provide compile-time verification of queries. This not only reduces the chances of runtime errors but also enhances developer confidence and productivity.
// Example of Type-Safe Query in Prisma
const user = await prisma.user.findUnique({
where: { id: 1 },
include: {
posts: true,
},
});
3. Database Agnostic:
Prisma is designed to be database agnostic, providing flexibility by supporting multiple databases, including PostgreSQL, MySQL, SQLite, and SQL Server. This allows developers to choose the database that best suits the requirements of their application without sacrificing the benefits of using Prisma.
4. Migrations and Schema Changes:
Prisma simplifies the process of database schema changes and migrations. With the prisma migrate
command, developers can easily manage and apply changes to the database schema, ensuring a smooth and organized evolution of the application’s data structure.
5. Prisma Client:
Prisma Client, an auto-generated query builder, acts as the bridge between your application code and the database. It provides a type-safe API for performing database operations, reducing the boilerplate code traditionally associated with database interactions.
// Example of Using Prisma Client
const newUser = await prisma.user.create({
data: {
name: 'John Doe',
email: 'john@example.com',
},
});
6. Scalability and Performance:
Prisma optimizes database queries, fetching only the data needed and minimizing unnecessary requests. This focus on efficiency contributes to the scalability and performance of backend applications, especially as they handle larger datasets and increasing user loads.
7. Real-Time Data with Prisma Client Realtime:
Prisma extends its capabilities to real-time data synchronization using Prisma Client Realtime. This feature allows applications to receive instantaneous updates when changes occur in the database, making it suitable for building real-time collaborative applications.
Conclusion:
Prisma stands out as a robust and feature-rich ORM for backend development, offering declarative data modeling, type-safe queries, database agnosticism, migrations, and a powerful Prisma Client. Its emphasis on efficiency, scalability, and developer-friendly features positions Prisma as a valuable tool for building modern and maintainable backend systems. As you embark on your backend development endeavors, consider incorporating Prisma to elevate your database interactions to new heights. Happy coding!